Animation
These experiments were made with THREE.js for the rendering course at the University Paul Sabatier in 2017.
Skeleton
We handle bones with a custom class even if THREE.js already provides one.
Our requirements for a bone are:
- A bone has a sphere of influence and it decreases with the distance
- Hierarchical bones, each one has a parent except the first one
- A vertex can be affected by two bones
- A debug interface should be provided to show area of influence and parent of a bone
Finally, a constructor can be called to create our bone. It takes in entry the position, the radius of the sphere of influence, its parent and a color for debug purposes.
Because of the implementation and automation of the computing of the area of influence, if one would want to have a more classical bone API, they would have to create two bones. The first one would be the kneecap and the second the area of influence at a fixed distance. The simple example Figure 1, uses this hierachy with 3 bones created and two visible in debug.
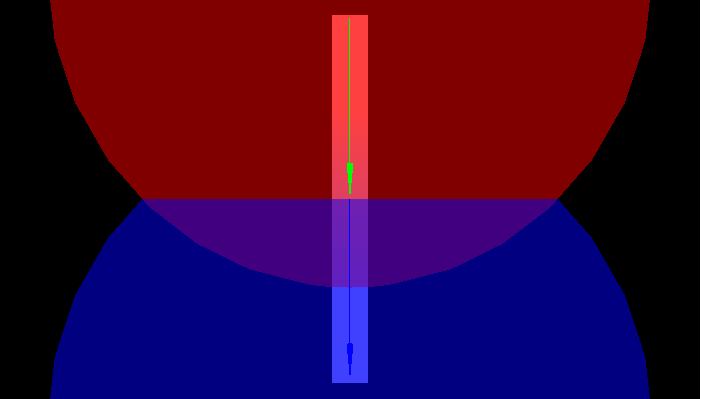
Rotation matrix and Quaternion
JavaScript is not really fast, so we immediately move calculation for blending onto the GPU. The code of the shader is exposed in the example at the end of this section.
We implement two way of representing rotations and transaltions, with rotation matrices and with dual quaternions. Both methods have their advantages and flaws. A linear blending is then applied with two bones (in our case, up to four could be handled but it generated uneasthetic results).
Rotation matrix blending creates a crushing at the point of rotation. It is like a folded straw Figure 2, and can be problematic when animating character with large limbs.
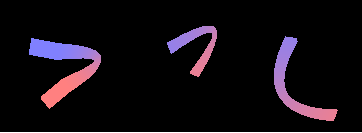
Dual quaternions blending creates a bulge around the point of rotation as shown on Figure 3.
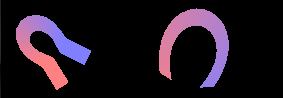
One of the main advantage of the duals quaternions linear blending is the handling of wringing. Whereas for rotation matrices we can spot a thinning of the volume during wringin, there is nothing like it with dual quaternions and the surface naturally roll up around the axis of rotation as shown in Figure 4.
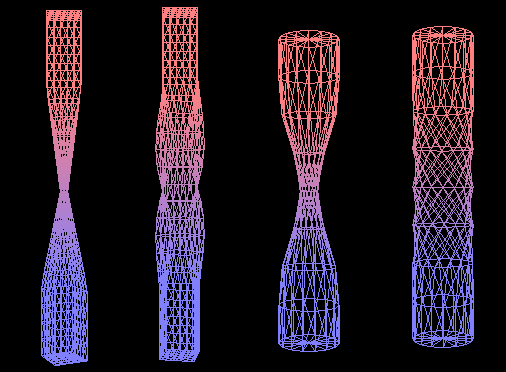
(Cuboid and cylinder with linear blending of matrices and dual quaternions)
All these configurations can be reproduced on the online example : Interactive example
Use case of animating a character with a hierarchical skeleton
We set up a hierarchical skeleton in order to animate “Marvin” our character. We use the rest position to place and create bones thanks to the debug mode Figure 5. We can then animate thanks to rotation transformations only so as to not distend the model.
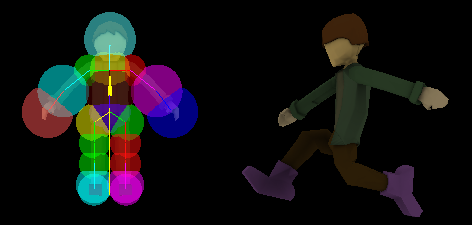
Animation can be visualized online : Animation of marvin